Have you ever wondered how companies like OpenAI or Google ensure their chatbots don't go off the rails? Welcome to the world of Large Language Model (LLM) evaluation – a crucial yet often overlooked aspect of AI development. In this post, we'll dive into the nuts and bolts of LLM evaluation, equipping you with the knowledge to assess and improve these powerful language models.
Understanding LLM Evaluation
What Is LLM Evaluation?
Picture this: You've just built an amazing AI chatbot for customer service. It's witty, it's fast, but… is it helpful? That's where LLM evaluation comes in. It's not just about making sure your model can string words together – it's about ensuring it's a reliable, fair, and effective tool for its intended purpose.
Why Is LLM Evaluation Important?
LLM evaluation isn't just for the scientists and programmers. It's crucial for anyone working with or relying on large language models. Here's why:
- Performance Boost: Find out where your model shines and where it needs a little TLC.
- Bias Busting: Catch those sneaky biases before they cause real-world problems.
- Safety First: Make sure your AI isn't going to say something it (and you) might regret.
- Stay Legal: Keep your model in line with industry regulations.
- Happy Users: Deliver top-notch results that'll keep your users coming back for more.
Key Metrics: How Do We Measure LLM Performance?
To measure how well your LLM is doing, consider these essential metrics:
Perplexity: The "Huh?" Factor
Perplexity is like measuring how often your model goes "Huh?" when trying to predict the next word. The lower the perplexity, the more confident your model is. Here's a quick way to calculate it:
import torch
from transformers import GPT2LMHeadModel, GPT2Tokenizer
model_name = 'gpt2'
model = GPT2LMHeadModel.from_pretrained(model_name)
tokenizer = GPT2Tokenizer.from_pretrained(model_name)
def calculate_perplexity(text):
encodings = tokenizer(text, return_tensors='pt')
max_length = model.config.n_positions
stride = 512
nlls = []
for i in range(0, encodings.input_ids.size(1), stride):
begin_loc = max(i + stride - max_length, 0)
end_loc = i + stride
trg_len = end_loc - i
input_ids = encodings.input_ids[:, begin_loc:end_loc]
target_ids = input_ids.clone()
target_ids[:, :-trg_len] = -100
with torch.no_grad():
outputs = model(input_ids, labels=target_ids)
neg_log_likelihood = outputs.loss * trg_len
nlls.append(neg_log_likelihood)
ppl = torch.exp(torch.stack(nlls).sum() / end_loc)
return ppl.item()
text = "The quick brown fox jumps over the lazy dog."
print("Perplexity:", calculate_perplexity(text))
🔑 Pro Tip: A lower perplexity score is generally better, but be cautious – a model that's too confident might be overfitting!
BLEU Score: The Translator
If your model is playing translator, BLEU is your go-to metric. It's like a game of "spot the difference" between your model's translation and a human reference. Here's how to use it:
from nltk.translate.bleu_score import sentence_bleu
reference = [['the', 'quick', 'brown', 'fox']]
hypothesis = ['the', 'fast', 'brown', 'fox']
score = sentence_bleu(reference, hypothesis)
print("BLEU Score:", score)
🌎 Real-world Example: When Google Translate improved its BLEU score by 2 points, it led to a noticeable improvement in translation quality for millions of users!
ROUGE Score: Judging Your AI's Summarization Skills
Ever tried to summarize a long article in a tweet? That's essentially what we're measuring with ROUGE (Recall-Oriented Understudy for Gisting Evaluation). It's like grading your AI's book report skills. ROUGE compares your AI's summary to a human-written one, checking for word overlap. It's like playing "Spot the Difference," but with words. Here's a quick way to calculate it:
from rouge import Rouge
hypothesis = "The quick brown fox jumps over the lazy dog."
reference = "A fast brown fox leaps over a lazy dog."
rouge = Rouge()
scores = rouge.get_scores(hypothesis, reference)
print("ROUGE Scores:", scores)
Fun Fact: ROUGE was originally developed for evaluating machine translation, but it found its true calling in summarization tasks.
Exact Match and F1 Score: The Dynamic Duo of Question Answering
If your AI is playing Jeopardy!, Exact Match (EM) and F1 Score are the judges.
Exact Match: The Perfectionist
Exact Match is exactly what it sounds like – the AI's answer needs to match the correct answer, word for word. It's binary: you either nailed it or you didn't.
def exact_match(prediction, ground_truth):
return int(prediction == ground_truth)
ai_answer = "42"
correct_answer = "42"
print("Exact Match:", exact_match(ai_answer, correct_answer))
F1 Score: The Flexible Friend
F1 Score is a bit more forgiving. It looks at the overlap between the predicted answer and the correct one. It's like giving partial credit on a test.
def f1_score(prediction, ground_truth):
pred_tokens = prediction.split()
truth_tokens = ground_truth.split()
common = set(pred_tokens) & set(truth_tokens)
if not common:
return 0
precision = len(common) / len(pred_tokens)
recall = len(common) / len(truth_tokens)
return 2 * (precision * recall) / (precision + recall)
ai_answer = "The capital of France is Paris"
correct_answer = "Paris is the capital of France"
print("F1 Score:", f1_score(ai_answer, correct_answer))
🔑 Pro Tip: Combine EM and F1 for a more balanced view. EM tells you how often your AI hits the bullseye, while F1 shows how close it gets when it misses.
Detecting and Mitigating Bias
AI bias isn't just a tech problem – it's a people problem. When AI systems reflect and amplify human biases, it can lead to unfair treatment, reduced opportunities, and reinforcement of societal stereotypes. And let's face it, nobody wants their AI to be that one awkward relative at Thanksgiving dinner who says all the wrong things.
Demographic Parity
Imagine you're throwing a party, and you want to make sure everyone gets an equal shot at the karaoke machine. That's essentially what Demographic Parity does – it checks if your AI is giving equal opportunities across different groups.
def demographic_parity(predictions, protected_attribute):
groups = set(protected_attribute)
group_rates = {g: sum(p for p, a in zip(predictions, protected_attribute) if a == g) / sum(a == g for a in protected_attribute) for g in groups}
return max(group_rates.values()) - min(group_rates.values())
# Example usage
predictions = [1, 0, 1, 1, 0, 1] # 1 for positive outcome, 0 for negative
protected_attribute = ['A', 'B', 'A', 'B', 'A', 'B'] # A and B are different groups
print(f"Demographic Parity Difference: {demographic_parity(predictions, protected_attribute)}")
A score close to 0 means your AI is being fair. If it's higher, well, your AI might be playing favorites.
Equal Opportunity
This metric checks if your AI is giving qualified candidates equal chances, regardless of their background. It's like making sure everyone gets a turn at karaoke, not just your tone-deaf buddy who really loves Beyoncé.
def equal_opportunity(predictions, protected_attribute, true_labels):
groups = set(protected_attribute)
group_rates = {g: sum(p for p, a, t in zip(predictions, protected_attribute, true_labels) if a == g and t == 1) / sum(a == g and t == 1 for a, t in zip(protected_attribute, true_labels)) for g in groups}
return max(group_rates.values()) - min(group_rates.values())
# Example usage
predictions = [1, 0, 1, 1, 0, 1] # 1 for positive outcome, 0 for negative
protected_attribute = ['A', 'B', 'A', 'B', 'A', 'B'] # A and B are different groups
true_labels = [1, 1, 1, 1, 0, 1] # 1 for actually qualified, 0 for not qualified
print(f"Equal Opportunity Difference: {equal_opportunity(predictions, protected_attribute, true_labels)}")
Again, closer to 0 is better.
Bias Detection Tools
- AI Fairness 360: IBM's open-source toolkit is like the Swiss Army knife of bias detection. It's got more metrics than you can shake a stick at, and it's prettier than your average command-line tool.
- Fairlearn: Microsoft's contribution to the fairness party. It's Python-based and plays nice with scikit-learn. Plus, it sounds like "fair learn," so you know it's good.
Other Evaluation Techniques
Once you've tackled bias, it's time to run your AI through the ultimate obstacle course. Here's the final trifecta of LLM evaluation:
Human Evaluation: Real people judging if your AI sounds human.
Task-Specific Assessment: Testing your AI on specific jobs. Can it translate, summarize, or code according to your business rules?
Real-World Scenario Testing: Throwing your AI into the wild. A/B testing, user feedback, and performance monitoring.
Comparing LLMs: Methodologies and Tools
The rapid advancement of Large Language Models (LLMs) has necessitated robust methods for their evaluation and comparison. This section explores the techniques and tools used to assess LLM performance, with a focus on comprehensive evaluation frameworks like jiant.
Comparing LLMs involves assessing their performance across various tasks and metrics. Popular models such as GPT-3, BERT, and T5 are often evaluated against each other to determine their strengths and weaknesses in different applications.
Top Methods for Evaluating Large Language Models
Evaluating Large Language Models (LLMs) is crucial for understanding their capabilities, limitations, and potential biases. Here are some of the top methods and tools used in the field:
Automated evaluations offer quick and repeatable assessments, allowing researchers and developers to consistently measure LLM performance across various tasks.
OpenAI's Evals Framework
OpenAI's Evals is a framework designed for systematic LLM evaluation. Key features include:
- Customizable evaluation scripts
- A registry of pre-defined evaluations
- Support for various model types, including OpenAI's models and custom implementations
- Ability to run evaluations on specific capabilities or general language understanding
Evals is particularly useful for those working with OpenAI's models or looking to create standardized evaluations for their own models.
EleutherAI's Language Model Evaluation Harness
EleutherAI's Evaluation Harness is a comprehensive framework for evaluating LLMs across a wide range of tasks. Notable aspects include:
- Support for numerous language models and tokenizers
- A large collection of NLP tasks and benchmarks
- Extensibility for adding custom tasks and models
- Detailed performance reporting and analysis tools
This harness is especially valuable for researchers looking to perform broad, multi-task evaluations of language models.
Hugging Face Datasets and Metrics
Hugging Face provides two key libraries for LLM evaluation:
- Offers easy access to a vast collection of NLP datasets
- Supports efficient data loading and processing
- Allows for easy sharing and versioning of datasets
- Provides a wide range of evaluation metrics for various NLP tasks
- Supports custom metric implementation
- Ensures consistent evaluation across different models and tasks
Additionally, Hugging Face maintains an Open LLM Leaderboard, which showcases performance comparisons of various open-source LLMs using standardized benchmarks. This leaderboard serves as a valuable resource for researchers and practitioners to assess state-of-the-art models.
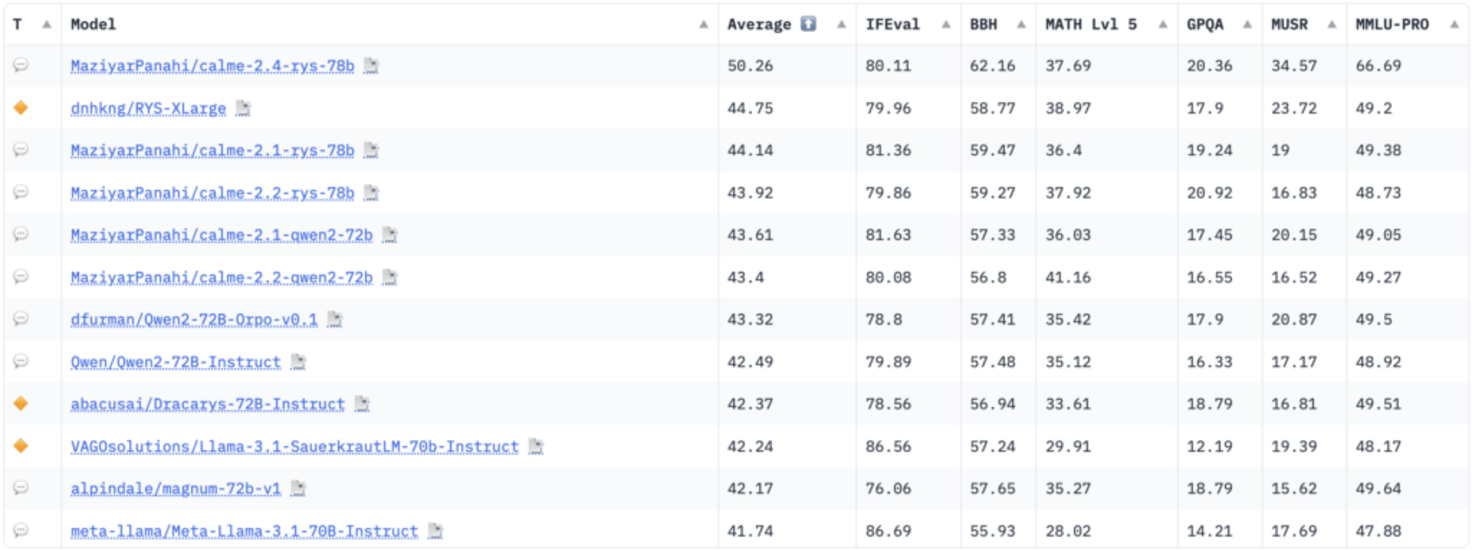
Stanford's HELM for LLM Evaluation
Stanford University's HELM (Holistic Evaluation of Language Models) offers another robust framework for comprehensive LLM assessment. HELM aims to provide a more nuanced and multifaceted evaluation of language models by considering a wide range of criteria beyond just performance metrics. It evaluates models across various dimensions including accuracy, calibration, robustness, fairness, bias, toxicity, and efficiency.
HELM's evaluation includes tests known as MMLU (Massive Multitask Language Understanding), a benchmark designed to assess language models across a wide range of human knowledge. MMLU covers 57 subjects, from humanities to STEM fields, testing both breadth and depth of understanding.
Building on this approach, HELM incorporates MMLU-style tests across various domains, including medicine. For example, in a medical knowledge assessment, GPT-4 was given a complex clinical scenario: Copy
A 47-year-old man with chest pain, dyslipidemia, hypertension, and diabetes presents with an ECG showing ST-segment elevation. After initial treatment, he collapses and becomes unresponsive. Which of the following is the most likely cause of death?
A) Papillary muscle rupture
B) Ventricular fibrillation
C) Septal wall rupture
D) Pulmonary embolism
GPT-4 correctly identified "Ventricular fibrillation" as the most probable cause, demonstrating its ability to synthesize complex medical information and apply clinical reasoning. This showcases HELM's capacity to assess not just factual recall, but also advanced problem-solving in specialized domains, extending beyond traditional MMLU tasks to evaluate real-world application of knowledge.
These libraries are particularly useful for researchers and practitioners who want to leverage standardized datasets and metrics in their evaluation pipelines.
Putting It All Together
Remember, no single metric tells the whole story. Effective LLM evaluation typically involves a combination of these methods, tailored to the specific use case and requirements of the model. By leveraging automated benchmarks, human evaluation, task-specific assessments, and real-world testing, researchers and practitioners can gain a comprehensive understanding of an LLM's capabilities and limitations.
As the field of LLM development continues to evolve rapidly, staying updated with the latest evaluation techniques and tools is crucial for ensuring the responsible development and deployment of these powerful language models.